Entrypoints โ
Standard entrypoints โ
FA2 library provides the 3 standard entrypoints: transfer
, balance_of
and update_operators
.
Their logic can be tweaked using the policies or replaced by introducing custom entrypoints.
transfer
โ
sp.transfer(<batch>)
The transfer
entrypoint takes a list of batches. Each batch is a record of:
from_
: the sender of the transfer.txs
: a list of record of:to_
: receiver of the transaction.token_id
: the token id.amount
: amount to be transferred.
Example:
c1.transfer(
[
sp.record(
from_ = alice.address,
txs = [
sp.record(to_=bob.address, amount=10, token_id=0),
sp.record(to_=bob.address, amount=10, token_id=1)
]
),
sp.record(
from_ = bob.address,
txs = [
sp.record(to_=alice.address, amount=11, token_id=0)
]
)
]).run(sender = charlie)
Types:
batch
:sp.TList(sp.TRecord(from_=sp.TAddress,ย txs=sp.TList(sp.TRecord(to_=sp.TAddress,ย token_id=sp.TNat,ย amount=sp.TNat).layout(("to_",ย ("token_id",ย "amount"))))).layout(("from_",ย "txs")))
Raises: FA2_TOKEN_UNDEFINED
, FA2_NOT_OPERATOR
, FA2_INSUFFICIENT_BALANCE
or FA2_TX_DENIED
.
Code documentation
transfer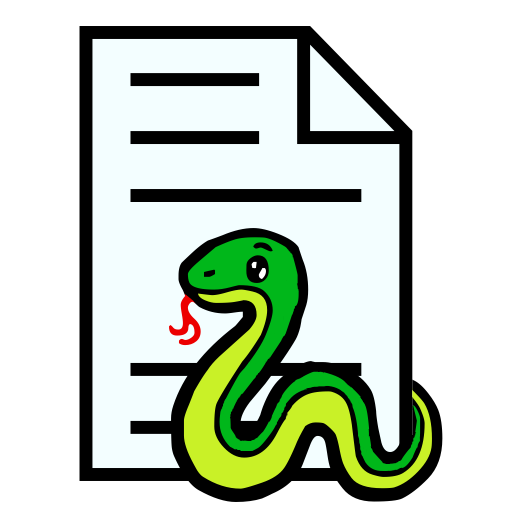
update_operators
โ
sp.update_operators(<batch>)
Operators are accounts that can transfer tokens on behalf of the owner.
The update_operators
entrypoint takes a list of batches. Each batch is a variant: "remove_operator"
or "add_operator"
. The value is a record of:
owner
: the account on which the operator can perform the transfer.operator
: the account to which the permission is granted.token_id
: the token_id on which the permission is granted.
Example:
c1.update_operators([
sp.variant("remove_operator", c1.operator_param.make(
owner = alice.address,
operator = op1.address,
token_id = 0)),
sp.variant("add_operator", c1.operator_param.make(
owner = alice.address,
operator = op2.address,
token_id = 0))
]).run(sender = alice)
Types:
batch
:sp.TList(sp.TVariant(add_operator=sp.TRecord(owner=sp.TAddress,ย operator=sp.TAddress,ย token_id=sp.TNat).layout(("owner",ย ("operator",ย "token_id"))),ย remove_operator=sp.TRecord(owner=sp.TAddress,ย operator=sp.TAddress,ย token_id=sp.TNat).layout(("owner",ย ("operator",ย "token_id")))))
Raises: FA2_NOT_OWNER
or FA2_OPERATORS_UNSUPPORTED
.
Code documentation
update_operators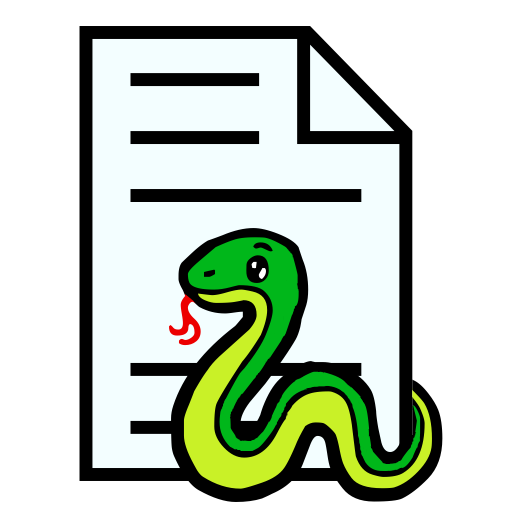
balance_of
โ
sp.balance_of(<callback>,ย <requests>)
Send the balance of multiple account / token pairs to a callback address.
Transfer 0 mutez to callback
with corresponding response.
Raises: FA2_TOKEN_UNDEFINED
, FA2_CALLBACK_NOT_FOUND
Types:
callback
:sp.TContract(sp.TList(sp.TRecord(request=sp.TRecord(owner=sp.TAddress,ย token_id=sp.TNat).layout(("owner",ย "token_id")),ย balance=sp.TNat).layout(("request",ย "balance"))))).layout(("requests",ย "callback"))
requests
:sp.TList(sp.TRecord(owner=sp.TAddress,ย token_id=sp.TNat).layout(("owner",ย "token_id")))
,
Example:
c1.balance_of(
callback=sp.contract(
sp.TList(t_balance_of_response),
c2.address,
entry_point="receive_balances",
).open_some(),
requests=[sp.record(owner=alice.address, token_id=0)],
).run(sender=alice)
Raises: FA2_NOT_OWNER
or FA2_OPERATORS_UNSUPPORTED
.
Code documentation
balance_of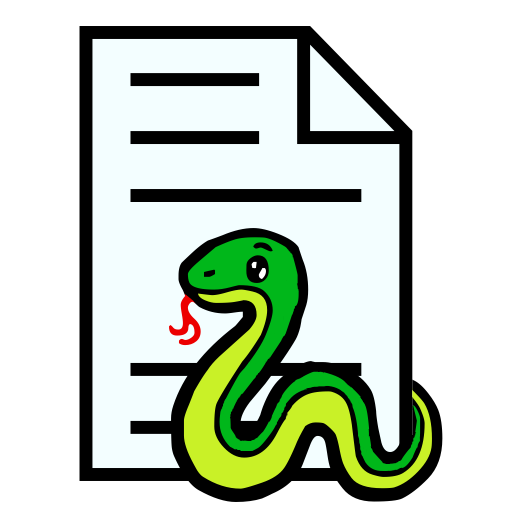
Custom entrypoints โ
You can reimplement the provided entrypoints or add your own ones exactly like you do in any SmartPy contracts. See entrypoints.
Example:
class Example_FA2(FA2.Fa2Fungible):
@sp.entrypoint
def my_entrypoint(self, params):
# ...
Non-standard entrypoints โ
The FA2 library provides non-standard entrypoints and views. They can be added using the mixins mechanism.
Mint and Burn โ
FA2 does NOT specify an interface for mint and burn operations as there can be a lot of different way to deal with them.
We provide mixins with these functionalities.
If you want to create your owns, it SHOULD, enforce the same logic as the transfer
entrypoint (core transfer behavior and transfer permission logic).
Mint and burn can be considered special cases of the transfer. Although, it is possible that mint and burn have more or less restrictive rules than the regular transfer. For instance, mint and burn operations may be invoked by a special privileged administrative address only. In this case, regular operator restrictions may not be applicable.